The verb to enqueue comes from the French language. It means to put something into a lineup.
In WordPress the wp_enqueue_style() and wp_enqueue_script() functions are used to manage the loading of external resources like scripts and stylesheets.
Why do we do things this way?
Imagine if you have a theme that requires a dependency (some other script or code library) to work. Imagine that in a site using your theme, the owner installs a plugin that requires jQuery or some other JavaScript library.
Several issues arise:
- Are you downloading jQuery twice?
- Are you downloading scripts in the right order?
- Are you downloading different, potentially incompatible, versions?
- Does the library load before or after other scripts?
- Do scripts in plugins and themes have identically named functions?
- Are all dependencies addressed?
- etc, etc
Ultimately, enqueuing styles and scripts allows WordPress to manage calls to these external resources and thus avoid a bunch of potential problems.
If a theme or plugin writer needs to use jQuery or some other library, they can specify this when they enqueue their scripts and WordPress will load its “official” version of the library.
For more detail, please read this page.
Enqueuing Some Google Fonts
Inside functions.php in the site is a function called rubberchicken_scripts_styles. Inside that at present is a single line that tells WordPress where to find the site’s stylesheet. It does this by calling a function that will return the absolute URL of the stylesheet.
Duplicate that line.
In the duplicated line, replace rubberchicken_style with rubberchicken_google_fonts.
Then replace the call to get_stylesheet_uri() with opening and closing quotation marks.
Now go to google fonts and select the following fonts:
- Goblin One
- Merriweather (weights 400, 400i, and 700)
In the EMBED part of the Google Fonts tab, select the URL that Google generates:
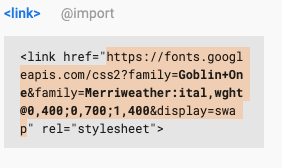
Back in functions.php, paste that value inside the quotation marks of our google fonts enqueueing function.
Reload the page. Open the Inspector and search for the word “Merriweather”.
Hopefully, you see this:

If you don’t, make sure that the “handle” given to the stylesheet is unique (ie make sure that you actually changed the first argument in the duplicated wp_enqueue_style function).
Summer 2020 note: Google has changed the format it uses for multiple font face or weight font downloads. This is causing only the first font family to be downloaded. To fix this, modify your enqueue statement as follows:
function rubberchicken_scripts_styles(){
wp_enqueue_style('rubberchicken_style', get_stylesheet_uri());
wp_enqueue_style('rubberchicken_google_fonts', 'https://fonts.googleapis.com/css2?family=Goblin+One&family=Merriweather:ital,wght@0,400;0,700;1,400&display=swap', array(), null);
Specifically, you need to add two additional parameters right at the end of the enqueue statement. More detail on this issue.
Note: you do not need to do this if you are enqueuing only one google font. In a pinch, if this does not work, you could also just use two enqueue statements (one for each font-family), although that would have a small effect on site performance.
About The Two Arguments We Passed
The HANDLE, the first argument, is a name for the stylesheet or script you wish to download.
The reason we need to give every requested script or stylesheet a handle is that it reduces the possibility of conflicts in naming. For WordPress to manage resources, each handle needs to be unique.
If you start with your theme name and add something unique for each script, it greatly increases the chances that your handle will not conflict with other scripts or stylesheets loaded by your theme, plugins, or WordPress itself, etc.
The Stylesheet (or Script) Location: next, we include the location of the stylesheet or script. In the original that we copied, the location of the stylesheet is inside the theme. As a result, we can dynamically generate the URL with just the get_stylesheet_uri() function.
With the google font download, however, we do not need to dynamically generate the URL because it is already absolute. In other words, we can replace the get_stylesheet_uri() part with just the location provided by google.
( Later, when we enqueue our own scripts, we’ll need to dynamically construct the URLs ).
LAB TASK 1:
Loading Font Awesome
Using the cdnjs ALL CSS Content Distribution Network Hosted Font Awesome, make Font Awesome load on your site. Use the first URL on that site (the minified all-css version).
To test if Font Awesome is loading, open the Chrome Inspector and search for the word awesome.
LAB TASK 2:
Social Icons for the Social Menu
Output the My Social Menu menu in bottom of the footer, below the secondary menu you put there earlier.
Now, figure out how to use the newly downloaded Font Awesome in your Social Menu.
When using Font Awesome 5, our font-family declaration depends on which Font Awesome collection you are using:
- Font Awesome Free: “Font Awesome 5 Free”
- Font Awesome Pro: “Font Awesome 5 Pro”
- Font Awesome Brands: “Font Awesome 5 Brands”
For the social icons, you want to use Font Awesome 5 Brands font-family.
Hint: How To Place the Icons
- because the menu is output by WordPress functions, you don’t have access to the html and therefore can’t use the traditional Font Awesome empty-span-or-i-with-a-class trick to create the icon.
- Instead, you will use the :before pseudo-class in your css to inject the icons into the document. Instructions for doing this can be found at the Font Awesome Documentation pages.
- you will need to use attribute selectors that select based on the LINKS’ HREF values
- for the before pseudo-class content, you will need to get the Unicode value for each icon. You can get these at the Font Awesome site. See below:
With each icon, the unicode value is found at the top. For example, in the SOUP icon below, the unicode value is f823.
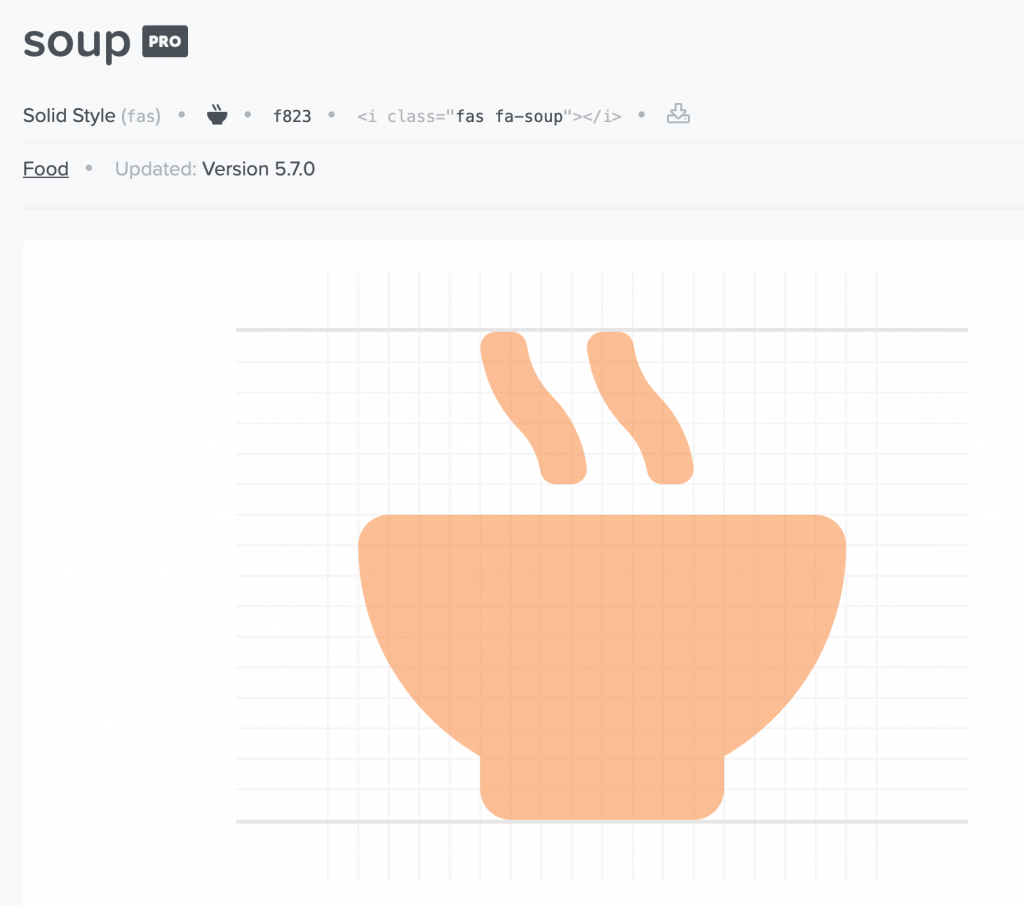
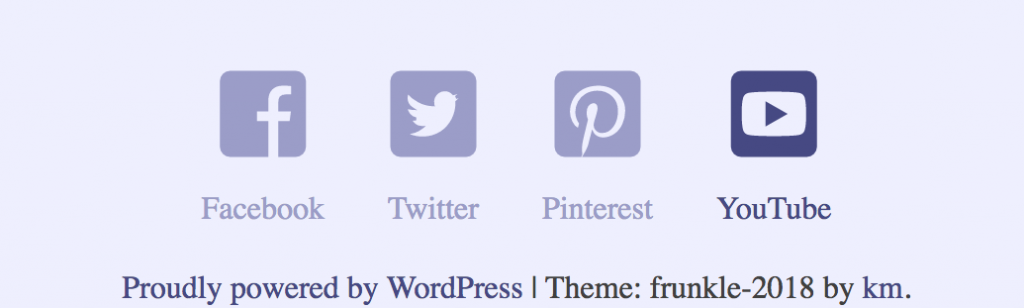
Finally, use the visual-hidden accessibility css to hide the words associated with each icon. This will require editing the code you have put into footer.php and consulting the WordPress Code Reference for wp_nav_menu. You can get the visually-hidden code from here.
In the screenshot below, we see see the link text is now not visible, while still remaining accessible to screenreaders.
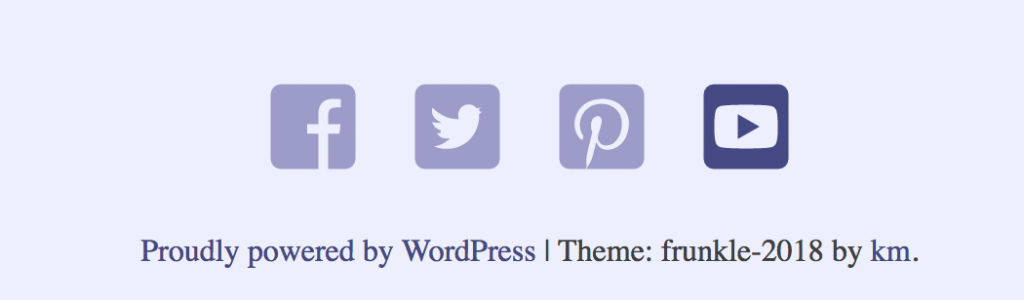
From an earlier version of this exercise, we see the social icons are set to not have the text. With CSS rather than additional classes, they’re styled with circles etc.
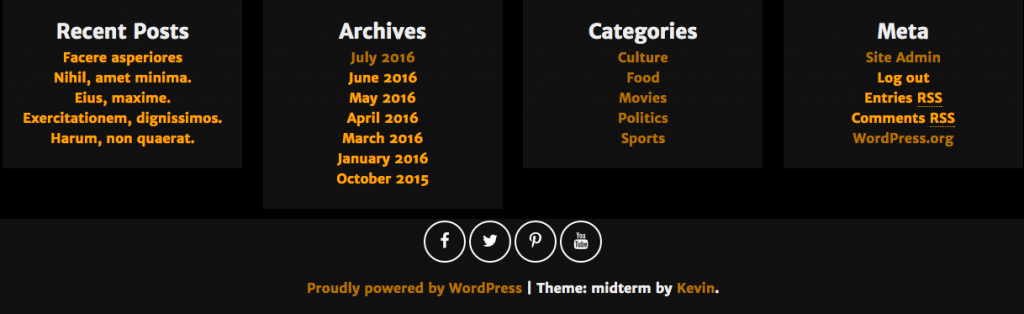
Lab Task 3: Strengthen Your CSS Selectors
Make sure that your selectors target all possible variations of the URLs of the social sites:
- https://facebook.com/etc
- https://facebook.com/etc
- https://www.facebook.com/etc
- https://www.facebook.com/etc
Make sure, also, that they are not so general that they insert icons in front of other phrases elsewhere in the site containing the word facebook, twitter, etc.
A useful catalog of possible CSS selectors to help in this task (particularly items 10 and 13).
Lab Task 4: Style the Site
Style the site so that every page is attractive and usable. Pay close attention to typography and responsive states.
Lab Task 5: Enqueue a Script
Figure out how to load a simple JavaScript that sends a message to the console on page load. Instructions are in part three of this exercise.